#Create New Invoice API
The following endpoint allows merchants to create a new invoice in their Fatora Platform account.
There are two way to add an invoice:
- Quick Invoice
- Detailed Invoice
Quick Invoice:
To add an invoice quickly by simply entering the amount and a description of the invoice.https://api.fatora.io/v1/invoices
curl -X POST "https://api.fatora.io/v1/invoices" \
--header "Authorization: Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1bmlxdWVfbmFtZSI6IjcyOTEyLTpyYW1pcmVzc2xhbkBnbWFpbC5jb20iLCJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9oYXNoIjoiOTExM2Y2OWEtMTYzMi00Nzg2LWE4NTctYmEzNWQ5YmEwMDc0IiwibmJmIjoxNzE5OTI0NDkzLCJleHAiOjE3NTEwMjg0OTMsImlhdCI6MTcxOTkyNDQ5M30.-rP1DT8LKk5jvBT_PpvaqFuUf_H6FhFiqAq9FM-34cw" \
--header "Content-Type: application/json" \
-d '{
"amount": 100.0,
"description": "Sample invoice description"
}'
// Define the API endpoint URL.
$apiUrl = "https://api.fatora.io/v1/invoices";
$invoice = [
"amount" => 100.0,
"description" => "Sample invoice description"
];
// Convert the data array to a JSON string.
$jsonPayload = json_encode($invoice);
// Initialize cURL session.
$ch = curl_init();
// Set cURL options.
curl_setopt($ch, CURLOPT_URL, $apiUrl);
curl_setopt($ch, CURLOPT_HTTPHEADER, ['Authorization: Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1bmlxdWVfbmFtZSI6IjcyOTEyLTpyYW1pcmVzc2xhbkBnbWFpbC5jb20iLCJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9oYXNoIjoiOTExM2Y2OWEtMTYzMi00Nzg2LWE4NTctYmEzNWQ5YmEwMDc0IiwibmJmIjoxNzE5OTI0NDkzLCJleHAiOjE3NTEwMjg0OTMsImlhdCI6MTcxOTkyNDQ5M30.-rP1DT8LKk5jvBT_PpvaqFuUf_H6FhFiqAq9FM-34cw', "Content-Type: application/json"]);
curl_setopt($ch, CURLOPT_POST, 1);
curl_setopt($ch, CURLOPT_POSTFIELDS, $jsonPayload);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the cURL session and capture the response.
$response = curl_exec($ch);
// Check for cURL errors.
if (curl_errno($ch)) {
echo "cURL Error: " . curl_error($ch);
}
// Close the cURL session.
curl_close($ch);
// Display the response.
echo "API Response: " . $response;
// Define the API endpoint URL.
string apiUrl = "https://api.fatora.io/v1/invoices";
var invoice = new Invoice
{
amount = 100.0m,
description = "Sample invoice description"
};
// Serialize the Product object to JSON.
string jsonPayload = JsonConvert.SerializeObject(invoice);
// Create a WebRequest.
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(apiUrl);
// Set the request method to POST.
request.Method = "POST";
request.Headers.Add("Authorization", "Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1bmlxdWVfbmFtZSI6IjcyOTEyLTpyYW1pcmVzc2xhbkBnbWFpbC5jb20iLCJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9oYXNoIjoiOTExM2Y2OWEtMTYzMi00Nzg2LWE4NTctYmEzNWQ5YmEwMDc0IiwibmJmIjoxNzE5OTI0NDkzLCJleHAiOjE3NTEwMjg0OTMsImlhdCI6MTcxOTkyNDQ5M30.-rP1DT8LKk5jvBT_PpvaqFuUf_H6FhFiqAq9FM-34cw");
// Set the request content type.
request.ContentType = "application/json";
// Convert the JSON payload to bytes.
byte[] data = Encoding.UTF8.GetBytes(jsonPayload);
// Set the content length.
request.ContentLength = data.Length;
// Get the request stream and write the JSON data to it.
using (var stream = request.GetRequestStream())
{
stream.Write(data, 0, data.Length);
}
try
{
// Get the response.
using (HttpWebResponse response = (HttpWebResponse)request.GetResponse())
{
if (response.StatusCode == HttpStatusCode.Created)
{
// Read the response data.
using (var responseStream = response.GetResponseStream())
using (var reader = new StreamReader(responseStream))
{
string responseContent = reader.ReadToEnd();
Console.WriteLine("API Response: " + responseContent);
}
}
else
{
Console.WriteLine("API Request failed with status code: " + response.StatusCode);
}
}
}
catch (WebException ex)
{
Console.WriteLine("API Request failed with an exception: " + ex.Message);
}
{
"status": "SUCCESS",
"invoice": {
"id": 2075,
"number": "INV567",
"currency": "QAR",
"terms_conditions": "Sample terms and conditions",
"note": null,
"date": "2023-10-24T10:21:00.9047758+02:00",
"status": "paid",
"type": "Detailed invoice",
"client": {
"id": 1307,
"name": "Client name",
"address": "Client address",
"phone": "Phone number",
"email": "[email protected]",
"notes": null
},
"title": "Sample Invoice",
"items": [
{
"id": 2376,
"product_id" : 123,
"description": "Sample invoice description",
"type": "Service Item",
"qty": 1.0,
"amount": 50.0
},
{
"id": 8796,
"product_id" : 456,
"description": "Sample invoice description",
"type": "Service Item",
"qty": 1.0,
"amount": 50.0
}
],
"payments": [
{
"id": 942,
"payment_date": "2023-10-10T00:00:00",
"transaction_id": "TXN123",
"amount": 50.0,
"note": "external",
"payment_type": "Credit",
"status": "Paid"
},
{
"id": 943,
"payment_date": "2023-10-10T00:00:00",
"transaction_id": "TXN456",
"amount": 50.0,
"note": "external",
"payment_type": "PayPal",
"status": "Paid"
}
],
"setting": {
"tax": true,
"discount": 0.0,
"discount_type": "",
"language": "ar",
"accepted_payment_methods": {
"card": true,
"bank_transfer": true,
"cash": true,
"paypal": true,
"e_signature": true
}
}
}
}
Try it now: Add Quick Invoice API
Detailed Invoice:
to add a comprehensive invoice by providing all related details, including invoice items, payments, currency, and more.curl -X POST "https://api.fatora.io/v1/invoices" \
--header "Authorization: Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1bmlxdWVfbmFtZSI6IjcyOTEyLTpyYW1pcmVzc2xhbkBnbWFpbC5jb20iLCJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9oYXNoIjoiOTExM2Y2OWEtMTYzMi00Nzg2LWE4NTctYmEzNWQ5YmEwMDc0IiwibmJmIjoxNzE5OTI0NDkzLCJleHAiOjE3NTEwMjg0OTMsImlhdCI6MTcxOTkyNDQ5M30.-rP1DT8LKk5jvBT_PpvaqFuUf_H6FhFiqAq9FM-34cw" \
--header "Content-Type: application/json" \
-d '{
"number": "INV123",
"currency": "QAR",
"terms_conditions": "Sample terms and conditions",
"note": "Sample note",
"date": "2023-09-30T00:00:00Z",
"status": "paid",
"type": "detailed",
"title": "Sample Invoice",
"client": {
"name": "Client name",
"email": "[email protected]",
"phone": "Phone number",
"address": "Client address"
},
"items": [
{
"product_id": "123",
"description": "Item 1",
"qty": 2,
"amount": 50.0
},
{
"product_id": "456",
"description": "Item 2",
"qty": 5,
"amount": 50.0
}
],
"payments": [
{
"payment_date": "2023-10-10T00:00:00Z",
"transaction_id": "TXN123",
"amount": 50.0,
"note": "Payment received",
"payment_type": "credit",
"status": "paid
}
],
"setting": {
"tax": true,
"discount": 10.0,
"discount_type": "Percentage",
"language": "ar",
"accepted_payment_methods": {
"card": true,
"bank_transfer": true,
"cash": true,
"paypal": true,
"e_signature": true
}
}
}'
// Define the API endpoint URL.
$apiUrl = "https://api.fatora.io/v1/invoices";
$invoice = [
"number" => "INV567",
"amount" => 100.0,
"currency" => "QAR",
"terms_conditions" => "Sample terms and conditions",
"note" => "Sample note",
"date" => date('Y-m-d\TH:i:s\Z'), // Format the date as required
"status" => "paid",
"type" => "detailed",
"title" => "Sample Invoice",
"description" => "Sample invoice description",
"client" => [
"name" => "Client name",
"email" => "[email protected]",
"phone" => "Phone number",
"address" => "Client address"
],
"items" => [
[
"product_id" => "123",
"description" => "Item 1",
"qty" => 2,
"amount" => 50.0
],
[
"product_id" => "456",
"description" => "Item 2",
"qty" => 5,
"amount" => 50.0
]
],
"payments" => [
[
"payment_date" => "2023-10-10T00:00:00Z",
"transaction_id" => "TXN123",
"amount" => 50.0,
"note" => "Payment received",
"payment_type" => "credit",
"status" => "paid"
]
],
"setting" => [
"tax" => true,
"discount" => 10.0,
"discount_type" => "Percentage",
"language" => "ar",
"accepted_payment_methods" => [
"card" => true,
"bank_transfer" => true,
"cash" => true,
"paypal" => true,
"e_signature" => true
]
]
];
// Convert the data array to a JSON string.
$jsonPayload = json_encode($invoice);
// Initialize cURL session.
$ch = curl_init();
// Set cURL options.
curl_setopt($ch, CURLOPT_URL, $apiUrl);
curl_setopt($ch, CURLOPT_HTTPHEADER, ['Authorization: Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1bmlxdWVfbmFtZSI6IjcyOTEyLTpyYW1pcmVzc2xhbkBnbWFpbC5jb20iLCJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9oYXNoIjoiOTExM2Y2OWEtMTYzMi00Nzg2LWE4NTctYmEzNWQ5YmEwMDc0IiwibmJmIjoxNzE5OTI0NDkzLCJleHAiOjE3NTEwMjg0OTMsImlhdCI6MTcxOTkyNDQ5M30.-rP1DT8LKk5jvBT_PpvaqFuUf_H6FhFiqAq9FM-34cw', "Content-Type: application/json"]);
curl_setopt($ch, CURLOPT_POST, 1);
curl_setopt($ch, CURLOPT_POSTFIELDS, $jsonPayload);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the cURL session and capture the response.
$response = curl_exec($ch);
// Check for cURL errors.
if (curl_errno($ch)) {
echo "cURL Error: " . curl_error($ch);
}
// Close the cURL session.
curl_close($ch);
// Display the response.
echo "API Response: " . $response;
// Define the API endpoint URL.
string apiUrl = "https://api.fatora.io/v1/invoices";
var invoice = new Invoice
{
number = "INV567",
amount = 100.0m,
currency = "QAR",
terms_conditions = "Sample terms and conditions",
note = "Sample note",
date = DateTime.Now,
status = "paid",
type = "detailed",
title = "Sample Invoice",
description = "Sample invoice description",
};
invoice.client = new Client
{
name = "Client name",
email = "[email protected]",
phone = "Phone number",
address = "Client address"
};
// Populate items
invoice.items = new List
{
new InvocieItem
{
"product_id" => "123",
description = "Item 1",
qty = 2,
amount = 50.0m
},
new InvocieItem
{
"product_id" => "456",
description = "Item 2",
qty = 5,
amount = 50.0m
}
};
// Populate payments
invoice.payments = new List
{
new InvoicePayment
{
payment_date = "2023-10-10",
transaction_id = "TXN123",
amount = 50.0m,
note = "Payment received",
payment_type = "credit",
status = "paid"
}
};
// Populate setting
invoice.setting = new InvoiceSetting
{
tax = true,
discount = 10.0m,
discount_type = "Percentage",
language = "ar",
accepted_payment_methods = new PaymentMethod
{
card = true,
bank_transfer = true,
cash = true,
paypal = true,
e_signature = true
}
};
// Serialize the Product object to JSON.
string jsonPayload = JsonConvert.SerializeObject(invoice);
// Create a WebRequest.
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(apiUrl);
// Set the request method to POST.
request.Method = "POST";
request.Headers.Add("Authorization", "Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1bmlxdWVfbmFtZSI6IjcyOTEyLTpyYW1pcmVzc2xhbkBnbWFpbC5jb20iLCJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9oYXNoIjoiOTExM2Y2OWEtMTYzMi00Nzg2LWE4NTctYmEzNWQ5YmEwMDc0IiwibmJmIjoxNzE5OTI0NDkzLCJleHAiOjE3NTEwMjg0OTMsImlhdCI6MTcxOTkyNDQ5M30.-rP1DT8LKk5jvBT_PpvaqFuUf_H6FhFiqAq9FM-34cw");
// Set the request content type.
request.ContentType = "application/json";
// Convert the JSON payload to bytes.
byte[] data = Encoding.UTF8.GetBytes(jsonPayload);
// Set the content length.
request.ContentLength = data.Length;
// Get the request stream and write the JSON data to it.
using (var stream = request.GetRequestStream())
{
stream.Write(data, 0, data.Length);
}
try
{
// Get the response.
using (HttpWebResponse response = (HttpWebResponse)request.GetResponse())
{
if (response.StatusCode == HttpStatusCode.Created)
{
// Read the response data.
using (var responseStream = response.GetResponseStream())
using (var reader = new StreamReader(responseStream))
{
string responseContent = reader.ReadToEnd();
Console.WriteLine("API Response: " + responseContent);
}
}
else
{
Console.WriteLine("API Request failed with status code: " + response.StatusCode);
}
}
}
catch (WebException ex)
{
Console.WriteLine("API Request failed with an exception: " + ex.Message);
}
{
"status": "SUCCESS",
"invoice": {
"id": 2075,
"number": "INV567",
"currency": "QAR",
"terms_conditions": "Sample terms and conditions",
"note": null,
"date": "2023-10-24T10:21:00.9047758+02:00",
"status": "paid",
"type": "Detailed invoice",
"client": {
"id": 1307,
"name": "Client name",
"address": "Client address",
"phone": "Phone number",
"email": "[email protected]",
"notes": null
},
"title": "Sample Invoice",
"items": [
{
"id": 2376,
"product_id" : 123,
"description": "Sample invoice description",
"type": "Service Item",
"qty": 1.0,
"amount": 50.0
},
{
"id": 8796,
"product_id" : 456,
"description": "Sample invoice description",
"type": "Service Item",
"qty": 1.0,
"amount": 50.0
}
],
"payments": [
{
"id": 942,
"payment_date": "2023-10-10T00:00:00",
"transaction_id": "TXN123",
"amount": 50.0,
"note": "external",
"payment_type": "Credit",
"status": "Paid"
},
{
"id": 943,
"payment_date": "2023-10-10T00:00:00",
"transaction_id": "TXN456",
"amount": 50.0,
"note": "external",
"payment_type": "PayPal",
"status": "Paid"
}
],
"setting": {
"tax": true,
"discount": 0.0,
"discount_type": "",
"language": "ar",
"accepted_payment_methods": {
"card": true,
"bank_transfer": true,
"cash": true,
"paypal": true,
"e_signature": true
}
}
}
}
Try it now: Add Detailed Invoice API
If you pass both an amount and a description along with items for the invoice, the amount and description will be used, and the items will be ignored. As a result, the invoice will be created as a quick invoice.
Header Parameters
Header | Value |
---|---|
Authorization: Bearer bearer_token
REQUIRED
|
obtain the bearer token from the Authorize endpoin. Read more information about Authentication. |
Content-Type
REQUIRED
|
application/json |
Request Body
Parameter | Description |
---|---|
description
REQUIREDIF: items parameter are not set
|
string the invoice details (limit of 300 characters). |
amount
REQUIREDIF: items parameter are not set
|
decimal The total amount of the invoice. |
date
OPTIONAL
|
string The date of the invoice. |
status
OPTIONAL
|
string The status of the invoice, The possible values are:"
["sent", "paid", "void", or "refund."]. by default it is sent. |
number
OPTIONAL
|
string A unique identifier for the invoice. |
currency
OPTIONAL
|
string The currency used for the invoice. Verify that the 'currency' value is a valid ISO currency code, and ensure that the specified 'currency' is supported by your account. If the provided currency is not in the list of currencies associated with your account, the API request will return a Bad Request error. |
client
OPTIONAL
|
object An object representing the client's details. It should
have the following properties:
|
note
OPTIONAL
|
string Additional notes related to the invoice (limit of 300 characters). |
type
OPTIONAL
|
string The accepted value for the type of the invoice is "detailed". Use this value to create a detailed invoice. If the 'type' is not set to 'detailed,' the API request will return a Bad Request error. |
terms_conditions
OPTIONAL
|
string Terms and conditions associated with the invoice (limit of 300 characters). |
items
REQUIREDIF: description and invoice amount are not set
|
array A list of invoice items. each representing a item for the
invoice.
Each item object should have the following properties:
|
payments
REQUIREDIF: status of invoice is set to paid or refund
|
array A list of payment objects, each representing a payment
for the invoice. - If the invoice status is "void" or "sent", payments must not be provided; otherwise, an error will occur. Each payment object should have the following properties:
|
setting
OPTIONAL
|
object an object representing the settings for an invoice. It
should have the following properties:
|
title
OPTIONAL
|
string The title or name of the invoice (limit of 300 characters). |
Response
Response Schema: application/json | |
---|---|
status
|
string the status of response. SUCCESS |
invoice
|
object:
|
Otherwise if there was a problem with your request, you'll receive an error response such as 400 status code, and the response will include an error object describing why this request failed.
Response Schema: application/json | |
---|---|
status
|
string The status of response. |
error
|
object:
|
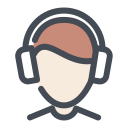
🛠️ Technical Support
- if you need any help you can check our help center, or contact support team