#Generate Product Image API
The following endpoint enables user to dynamically create contextually relevant product images using.
https://api.fatora.io/ai/generateImage
curl --location 'https://api.fatora.io/ai/generateImage' \
--header 'Content-Type: application/json' \
--data-raw '{
"name": "Smartphone"
}'
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://api.fatora.io/ai/generateImage',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_POSTFIELDS => '{
"name": "Sample Product name"
}',
CURLOPT_HTTPHEADER => array(
'Content-Type: application/json'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
using System.IO;
using System.Net;
using System.Text;
class Program
{
static void Main()
{
string product_name = "your_product_name"; // Replace with the actual product name
// Prepare the data object
var data = new
{
name = product_name
};
// Convert data object to JSON
string jsonData = Newtonsoft.Json.JsonConvert.SerializeObject(data);
// API endpoint URL
string apiUrl = "https://api.fatora.io/ai/generateImage";
// Create HttpWebRequest
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(apiUrl);
request.Method = "POST";
request.ContentType = "application/json";
// Write JSON data to the request stream
using (StreamWriter streamWriter = new StreamWriter(request.GetRequestStream()))
{
streamWriter.Write(jsonData);
streamWriter.Flush();
streamWriter.Close();
}
try
{
// Get the response
using (HttpWebResponse response = (HttpWebResponse)request.GetResponse())
using (Stream stream = response.GetResponseStream())
using (StreamReader reader = new StreamReader(stream))
{
string responseText = reader.ReadToEnd();
Console.WriteLine(responseText);
}
}
catch (WebException ex)
{
if (ex.Response is HttpWebResponse webResponse)
{
Console.WriteLine($"Error: Request failed with status: {webResponse.StatusCode}");
}
else
{
Console.WriteLine($"Error: {ex.Message}");
}
}
}
}
{
"status": "SUCCESS",
"product": {
"image_urls": ["url1", "url2", "url3", "url4"]
}
}
Try it now: Generate Product Image API
Header Parameters
Header | Value |
---|---|
Content-Type
REQUIRED
|
application/json |
Request Body
Parameter | Description |
---|---|
name
REQUIRED
|
stringThe name of the product. |
Response
Response Schema: application/json | |
---|---|
status
|
string the status of response. SUCCESS |
product
|
object:
|
Otherwise if there was a problem with your request, you'll receive an error response such as 400 status code, and the response will include an error object describing why this request failed.
Response Schema: application/json | |
---|---|
status
|
string The status of response. |
error
|
object:
|
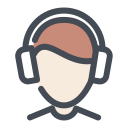
🛠️ Technical Support
- if you need any help you can check our help center, or contact support team