#Get Product API.
The following endpoint allows merchant to get product from their Fatora Platform account.
https://api.fatora.io/v1/products/{product_id}
curl --location --request GET 'https://api.fatora.io/v1/products/{product_id}' \
--header 'api_key: E4B73FEE-F492-4607-A38D-852B0EBC91C9' \
--header 'Content-Type: application/json' \
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://api.fatora.io/v1/products/{product_id}',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_HTTPHEADER => array(
'api_key: E4B73FEE-F492-4607-A38D-852B0EBC91C9',
'Content-Type: application/json'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
// Define the API endpoint URL.
string apiUrl = "https://api.fatora.io/v1/products/{product_id}";
// Define the API key.
string apiKey = "E4B73FEE-F492-4607-A38D-852B0EBC91C9";
// Create a WebRequest.
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(apiUrl);
// Set the request method to GET.
request.Method = "GET";
// Set the API key header.
request.Headers.Add("api_key", apiKey);
// Set the request content type.
request.ContentType = "application/json";
try
{
// Get the response.
using (HttpWebResponse response = (HttpWebResponse)request.GetResponse())
{
if (response.StatusCode == HttpStatusCode.OK)
{
// Read the response data.
using (var responseStream = response.GetResponseStream())
using (var reader = new StreamReader(responseStream))
{
string responseContent = reader.ReadToEnd();
Console.WriteLine("API Response: " + responseContent);
}
}
else
{
Console.WriteLine("API Request failed with status code: " + response.StatusCode);
}
}
}
catch (WebException ex)
{
Console.WriteLine("API Request failed with an exception: " + ex.Message);
}
{
"status": "SUCCESS",
"product": {
"id": 123,
"name": "Sample Item",
"price": 19.99,
"sku": "SKU123",
"image": "https://example.com/sample-image.jpg",
"description": "This is a sample item description.",
"creation_date": "2023-09-30T12:00:00",
"currency": "QAR",
"category": ["Category1", "Category2"],
"qr_code": "https://api.qrserver.com/v1/create-qr-code/?data=sampleQRcode&size=50x50",
"link": "https://example.com/sample-link",
"status": "Active",
"language": "en",
"setting": {
"is_public": true,
"is_limited": false,
"is_casheir": true,
"max_count": 100,
"max_order": 10
}
}
}
Try it now: Get Product API
Header Parameters
Header | Value |
---|---|
api_key
REQUIRED
|
Use the valid API key of your Fatora account. Read more information about Authentication. |
Content-Type
REQUIRED
|
application/json |
Query Parameters
Parameter | Description |
---|---|
product_id
REQUIRED
|
intUnique identifier of the product to retrieved. |
Response
HTTP Status Code: 200 OK - The product information has been successfully retrieved.
Response Schema: application/json | |
---|---|
status
|
string the status of response. SUCCESS |
product
|
object:
|
Otherwise if there was a problem with your request, you'll receive an error response such as 400 status code, and the response will include an error object describing why this request failed.
Response Schema: application/json | |
---|---|
status
|
string The status of response. |
error
|
object:
|
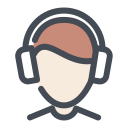
🛠️ Technical Support
- if you need any help you can check our help center, or contact support team