# Extract Invoice Info API
The following endpoint enables user to extract relevant invoice info (invoice number, invoice date, invoice total, invoice description) from pdf invoice file .
https://api.fatora.io/ai/extractInvoiceInfo
curl --location --request POST $API_ENDPOINT \
--header 'Content-Type: multipart/form-data' \
--header 'api_key: E4B73FEE-F492-4607-A38D-852B0EBC91C9' \
-F 'invoice_file=@'$PDF_FILE_PATH'
-F 'add_to_account="1"'
// API Endpoint
$apiEndpoint = "https://api.fatora.io/ai/extractInvoiceInfo";
// File to Upload
$pdfFilePath = "/path/to/your/invoice.pdf";
// Headers
$headers = [
'Content-Type: multipart/form-data',
'api_key: E4B73FEE-F492-4607-A38D-852B0EBC91C9',
];
// Initialize cURL session
$ch = curl_init();
// Set cURL options
curl_setopt($ch, CURLOPT_URL, $apiEndpoint);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, [
'invoice_file' => new CURLFile($pdfFilePath),
'add_to_account' => true
]);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
// Execute cURL session and get the response
$response = curl_exec($ch);
// Check for cURL errors
if (curl_errno($ch)) {
echo 'Curl error: ' . curl_error($ch);
}
// Close cURL session
curl_close($ch);
// Display the API response
echo $response;
using System;
using System.IO;
using System.Net.Http;
using System.Threading.Tasks;
class Program
{
static void Main()
{
Task.Run(async () => await YourAsyncMethod()).Wait();
}
static async Task YourAsyncMethod()
{
// Replace "your_invoice_file_path" with the actual file path
string filePath = "your_invoice_file_path";
await UploadFileAsync(filePath);
}
static async Task UploadFileAsync(string filePath)
{
try
{
// Define the API key.
string apiKey = "E4B73FEE-F492-4607-A38D-852B0EBC91C9";
// Create HttpClient
using (HttpClient client = new HttpClient())
using (MultipartFormDataContent form = new MultipartFormDataContent())
using (FileStream fileStream = File.OpenRead(filePath))
{
// Append the file to the form data
form.Add(new StreamContent(fileStream), "invoiceFile", Path.GetFileName(filePath));
// Add API key to the request header
client.DefaultRequestHeaders.Add("api_key", apiKey);
// API endpoint URL
string apiUrl = "https://api.fatora.io/ai/extractInvoiceInfo";
// Send the request
HttpResponseMessage response = await client.PostAsync(apiUrl, form);
// Handle the response
if (response.IsSuccessStatusCode)
{
Console.WriteLine("File uploaded successfully");
// Extract and handle the response text
string responseText = await response.Content.ReadAsStringAsync();
}
else
{
Console.WriteLine($"Error: Request failed with status: {response.StatusCode}");
}
}
}
catch (Exception ex)
{
Console.WriteLine($"Error: {ex.Message}");
}
}
}
{
"status": "SUCCESS",
"invoice": {
"invoice_number": "INV_123",
"invoice_date": "01/01/2024",
"invoice_price":"539.41",
"line_items": "items decription of invoice"
}
}
Try it now: Extract Invoice Info API
Header Parameters
Header | Value |
---|---|
api_key
REQUIRED
|
Use the valid API key of your Fatora account. Read more information about Authentication. |
Content-Type
REQUIRED
|
application/json |
Request Body
Parameter | Description |
---|---|
File
REQUIRED
|
fileThe pdf file of the invoice. |
add_to_account
OPTIONAL
|
boolIf you wish to associate the invoice with your account, please set this parameter to "true". |
Response
Response Schema: application/json | |
---|---|
status
|
string the status of response. SUCCESS |
invoice
|
object:
|
Otherwise if there was a problem with your request, you'll receive an error response such as 400 status code, and the response will include an error object describing why this request failed.
Response Schema: application/json | |
---|---|
status
|
string The status of response. |
error
|
object:
|
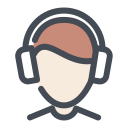
🛠️ Technical Support
- if you need any help you can check our help center, or contact support team