Integrate Fatora With Wix Store
You can integrate any wix website with Fatora, follow this steps.
Step1: Access to admin dashboard
-
Go to your website and loginto your account
-
Select the website which you want to add to it the payment gateway.
Step2: Set Up Manual Payments
-
From the left side menu in the dashboard, select settings then select the Accept payments.
-
Select Manual.
-
Click Connect Me.
-
Click Manage Manual Payments.
-
Click the drop-down menu and select a title for the payment instructions.
-
Enter the instructions in the text box (e.g. ⚠ You will be redirected to the Fatora payment page.).
Step3: Edit a Thank You Page
Adding Wix Stores automatically adds a new page, called Shop, to your site and 3 hidden pages- The Product page: Displayed when a customer clicks a product in your product gallery.
- The Cart page: Displayed when a customer clicks the Cart icon at the top, or the Add to Cart button on a product page.
- The Thank You page: Displayed when a customer makes a purchase.
-
From the left side menu in the dashboard, click on Edit Site
-
Click on Dev Mode, then click on Turn on DevMode
-
Click Thank You Page.
-
Edit Thank You Page, then add button with id = "btnPay".
-
Open code editor from the bottom of the thank you page, delete any code here, then add the following script code.
import wixUsers from 'wix-users';
import {generateLink} from 'backend/generateLink';
$w.onReady(() => {
$w('#btnPay').label = "⏱ Please wait for the payment URL...";
var payLink = "Not Set";
$w('#thankYouPage1').getOrder().then( (order) => {
var order_id = order.number,
client_name = order.billingInfo.firstName + " " + order.billingInfo.lastName,
client_email = order.billingInfo.email,
amount = order.totals.total,
currency = order.currency;
generateLink(amount,currency, order_id,client_email).then(result => {
payLink = result;
$w('#btnPay').link = result;
$w('#btnPay').label = "Pay "+ amount + " now 🛒";
// check if the user is logged in
if(wixUsers.currentUser.loggedIn) {
const userId = wixUsers.currentUser.id;
wixUsers.emailUser('ibhMail', userId, {
variables: {
"payURL": payLink,
"amount":amount.toString(),
"orderId":order_id.toString(),
"name":client_name.toString()
}});
}else // then let's use the email he used in the checkout and send him a payment link
{
const subject = "#" + client_email + "Payment Link";
const body = `
\rPayment Link: ${encodeURIComponent(payLink)}`;
}
}).catch(err => console.log(err));
});
});
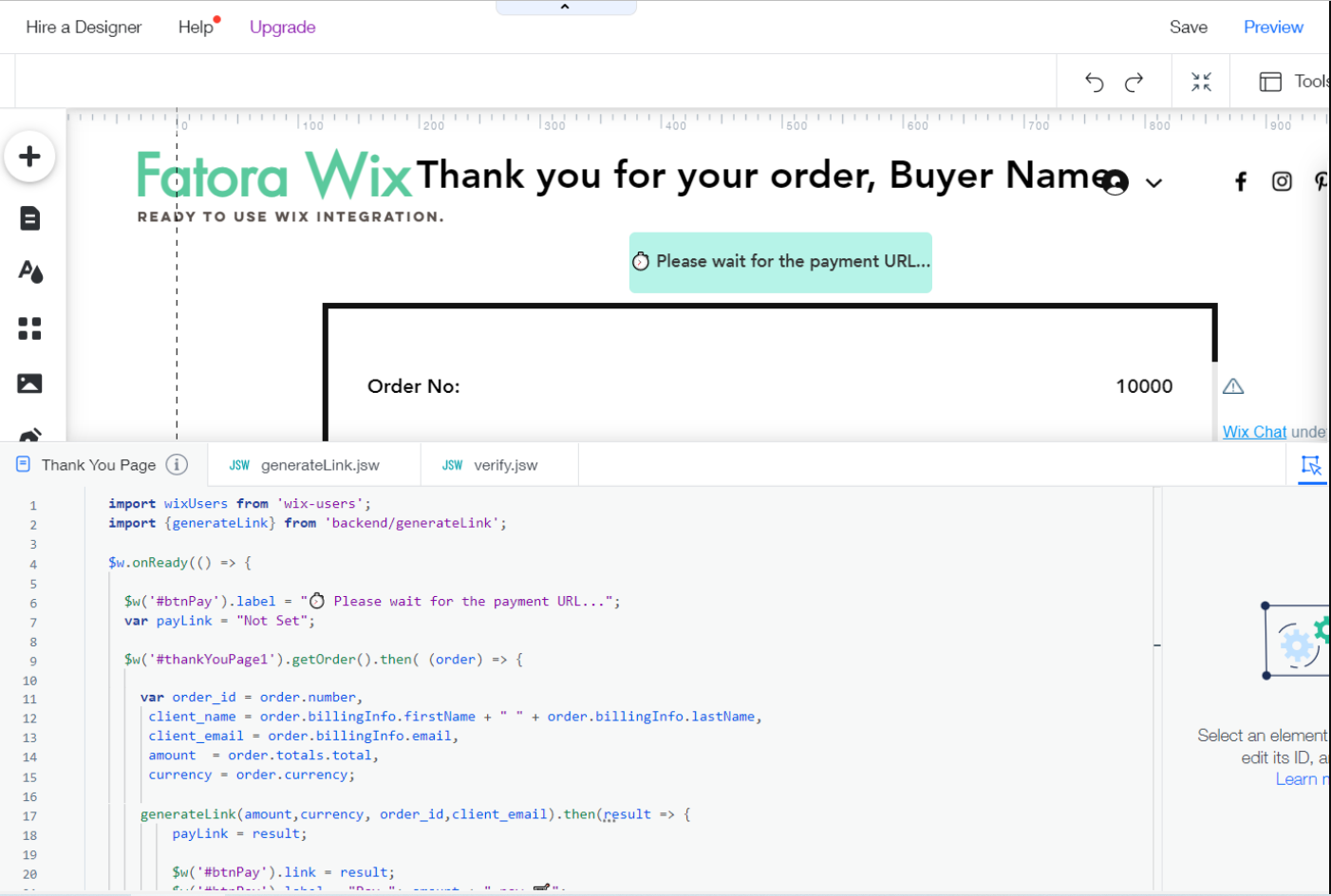
Be Attention!
- When you add button, you must be sure that the id of pay button is as the same as the id in
script code which it is here
"btnPay"
Setp4: Create module file for rquest Fatora standard checkout API endpoint
-
From sidebar click on Code Files, select Backend, then Create new web module file with name generateLink.jsw
-
Edit file and add the following script standard checkout api.
import { fetch } from 'wix-fetch';
import wixSite from 'wix-site-backend';
export function getUserName() {
return {
websiteEmail: wixSite.generalInfo.getEmail(),
websitePhone: wixSite.generalInfo.getPhone()
};
}
export function generateLink(amount, currency, order_id, client_email) {
const fatoraApi = "https://api.fatora.io/v1/payments/checkout";
return fetch(fatoraApi, {
method: 'post',
mode: 'cors',
body: JSON.stringify({
"amount": amount,
"currency": currency,
"order_id": order_id,
"client": {
"name": "client_name",
"phone": "+974XXXXXXXX",
"email": client_email,
},
"lang": "en",
"success_url": "http://domain.com/payments/success",
"failure_url": "http://domain.com/payments/failure",
"fcm_token": "XXXXXXXXX",
"save_token": false,
"note": "some note here",
}),
headers: {
"Content-Type": "application/json; charset=utf-8",
"api_key": "E4B73FEE-F492-4607-A38D-852B0EBC91C9"
}
})
.then(response => response.json())
.then(json => json.result.checkout_url);
}
Be Attention!
- Replace test
api_key
value with yours which you have in your account. - Replace
success_url
value with yours as you define in your site - Replace
failure_url
value with yours as you define in your site - Replace
lang
value with "ar" if you want payent page language in appear in arabic - Replace
client.name
&client.phone
with actual values
Step5: Create Completation Page (success, failure) page
When your client completes the payment, Fatora gateway will redirect to the default Fatora success | failure page, If you pass success_url | failure_url at requesting Standard Checkout API, Fatora will redirect after 2 seconds to the (success | failure) page. We recommend you to add one page for both (success | failure) pages and check the status of payment in this page and let it be called a complete page
-
Edit complete page and click on add button, Select multi box to add on complete page
-
Edit multi box and change the title of fields on Full status like this
- Set the title of field1 as Order ID, set the ID as "OrderIdTxt"
- Set the title of field2 as Email, set the ID as "emailTxt"
-
Open code editor from the bottom of the complete you page, delete any code here, and then add the following script code
// to get querd parameters from the url
import wixLocation from 'wix-location';
// back end call to validate the payment with fatora
import {validatePayment} from 'backend/verify.jsw';
// front end user func's to play with
import wixUsers from 'wix-users';
// back end call function to get the user nickname
import {getUserName} from 'backend/generateLink';
// Get Quered data from the URL
var query = wixLocation.query;
let order_id = query.order_id,
transaction_id = query.transaction_id;
// Validate The payment with Fatora
// When you ready let's change the world says by Pinky & The Brain
$w.onReady(() => {
validatePayment(order_id, transaction_id).then(paymentstate => {
// get the status of the payment
var status = paymentstate;
// change the multi status to full where there is content
$w("#fullEmpty").changeState("Full");
// Set text's at page
$w("#OrderIdTxt").text = order_id;
$w("#emailTxt").text = "[email protected]";
// Check if the payment were successfully paid & if the user us logged in and send an email to the user
if (status === "SUCCESS" && wixUsers.currentUser.loggedIn) {
// Send an email the client that the payment were successful
// call a promise after the value known and fulfilled.
getUserName().then( (results) => {
console.log(results);
wixUsers.emailUser('RlHBxh5', wixUsers.currentUser.id, {
variables: {
contactEmail: "[email protected]",
phoneNumber: "0597752790",
orderId: order_id
}});
});
}
}).catch((error) => console.log(error));
});
Setp6: Create module file for rquest Fatora Verify API endpoint
-
From sidebar click on Code Files, select Backend, then Create new web module file with name verify.jsw
-
Edit file and add the following script verify api.
import { fetch } from 'wix-fetch';
export function validatePayment(order_id, transaction_id) {
const fatoraApi = "https://api.fatora.io/v1/payments/verify";
return fetch(fatoraApi, {
method: 'post',
mode: 'cors',
body: JSON.stringify({
order_id: order_id,
transaction_id: transaction_id
}),
headers: {
"Content-Type": "application/json; charset=utf-8",
"api_key": "E4B73FEE-F492-4607-A38D-852B0EBC91C9"
}
})
.then(response => response.json())
.then(json => json.payment_status);
}
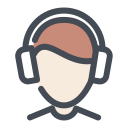
🛠️ Technical Support
- if you need any help you can check our help center, or contact support team