#Update Product Information API
The following endpoint allows merchant to update product information in their Fatora Platform account.
https://api.fatora.io/v1/products/{product_id}
curl --location 'https://api.fatora.io/v1/products/669' \
--request PUT \
--header "Authorization: Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1bmlxdWVfbmFtZSI6IjcyOTEyLTpyYW1pcmVzc2xhbkBnbWFpbC5jb20iLCJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9oYXNoIjoiOTExM2Y2OWEtMTYzMi00Nzg2LWE4NTctYmEzNWQ5YmEwMDc0IiwibmJmIjoxNzE5OTI0NDkzLCJleHAiOjE3NTEwMjg0OTMsImlhdCI6MTcxOTkyNDQ5M30.-rP1DT8LKk5jvBT_PpvaqFuUf_H6FhFiqAq9FM-34cw" \
--header 'Content-Type: application/json' \
--data-raw '{
"name": "Updated Item Name",
"price": 29.99,
"sku": "UpdatedSKU123",
"image": "https://example.com/updated-sample-image.jpg",
"description": "This is an updated item description.",
"language": "en",
"currency": "QAR",
"status": "Normal",
"category": "UpdatedCategory1",
"setting": {
"is_public": false,
"is_limited": true,
"is_casheir": false,
"max_count": 20,
"max_order": 5
}
}'
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://api.fatora.io/v1/products/669',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'PUT',
CURLOPT_POSTFIELDS => '{
"name": "Updated Item Name",
"price": 29.99,
"sku": "UpdatedSKU123",
"image": "https://example.com/updated-sample-image.jpg",
"description": "This is an updated item description.",
"language": "en",
"currency": "QAR",
"status": "Normal",
"category": "UpdatedCategory1",
"setting": {
"is_public": false,
"is_limited": true,
"is_casheir": false,
"max_count": 20,
"max_order": 5
}
}',
CURLOPT_HTTPHEADER => array(
'Authorization: Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1bmlxdWVfbmFtZSI6IjcyOTEyLTpyYW1pcmVzc2xhbkBnbWFpbC5jb20iLCJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9oYXNoIjoiOTExM2Y2OWEtMTYzMi00Nzg2LWE4NTctYmEzNWQ5YmEwMDc0IiwibmJmIjoxNzE5OTI0NDkzLCJleHAiOjE3NTEwMjg0OTMsImlhdCI6MTcxOTkyNDQ5M30.-rP1DT8LKk5jvBT_PpvaqFuUf_H6FhFiqAq9FM-34cw',
'Content-Type: application/json'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
// Define the API endpoint URL.
string apiUrl = "https://api.fatora.io/v1/products/669";
var product = new Product
{
name = "Updated Item Name",
code = "Product Code",
category = "UpdatedCategory1",
currency = "QAR",
status = "Normal",
language = "ar",
sku = "UpdatedSKU123",
price = 100.0,
image = "https://example.com/updated-sample-image.jpg",
description = "This is an updated item description.",
setting = new ProductSetting
{
is_casheir = false,
is_limited = true,
is_public = false,
max_count = 20,
max_order = 5
}
};
// Serialize the Product object to JSON.
string jsonPayload = JsonConvert.SerializeObject(product);
// Create a WebRequest.
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(apiUrl);
// Set the request method to PUT.
request.Method = "PUT";
request.AddHeader("Authorization", "Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1bmlxdWVfbmFtZSI6IjcyOTEyLTpyYW1pcmVzc2xhbkBnbWFpbC5jb20iLCJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9oYXNoIjoiOTExM2Y2OWEtMTYzMi00Nzg2LWE4NTctYmEzNWQ5YmEwMDc0IiwibmJmIjoxNzE5OTI0NDkzLCJleHAiOjE3NTEwMjg0OTMsImlhdCI6MTcxOTkyNDQ5M30.-rP1DT8LKk5jvBT_PpvaqFuUf_H6FhFiqAq9FM-34cw");
// Set the request content type.
request.ContentType = "application/json";
// Convert the JSON payload to bytes.
byte[] data = Encoding.UTF8.GetBytes(jsonPayload);
// Set the content length.
request.ContentLength = data.Length;
// Get the request stream and write the JSON data to it.
using (var stream = request.GetRequestStream())
{
stream.Write(data, 0, data.Length);
}
try
{
// Get the response.
using (HttpWebResponse response = (HttpWebResponse)request.GetResponse())
{
if (response.StatusCode == HttpStatusCode.OK)
{
// Read the response data.
using (var responseStream = response.GetResponseStream())
using (var reader = new StreamReader(responseStream))
{
string responseContent = reader.ReadToEnd();
Console.WriteLine("API Response: " + responseContent);
}
}
else
{
Console.WriteLine("API Request failed with status code: " + response.StatusCode);
}
}
}
catch (WebException ex)
{
Console.WriteLine("API Request failed with an exception: " + ex.Message);
}
{
"status":"SUCCESS",
"product":{
"id":669,
"name":"Product Name",
"price":102.0,
"sku":null,
"image":"https://domain.com/itm/ckk00242023-X5PBR.jpg",
"qr_code":"https://api.qrserver.com/v1/create-qr-code/?data=&size=50x50",
"description":"Product Description",
"setting":{
"is_public":false,
"is_limited":false,
"is_casheir":false,
"max_count":0.00,
"max_order":0
},
"creation_date":"2023-10-21T09:51:59.933",
"category":"Category1",
"language":"ar",
"currency":"QAR",
"link":"https://example.com/sample-link",
"status":"Normal"
}
}
Try it now: Update Product API
Header Parameters
Header | Value |
---|---|
Authorization: Bearer bearer_token
REQUIRED
|
obtain the bearer token from the Authorize endpoin. Read more information about Authentication. |
Content-Type
REQUIRED
|
application/json |
Query Parameters
Parameter | Description |
---|---|
product_id
REQUIRED
|
intUnique identifier of the product to update. |
Request Body
Parameter | Description |
---|---|
name
REQUIRED
|
stringThe name of the product. |
price
OPTIONAL
|
decimalThe price of the item. |
sku
OPTIONAL
|
stringStock Keeping Unit (SKU) code for the product. |
image
OPTIONAL
|
stringURL or base64-encoded image of the product. |
description
OPTIONAL
|
stringDescription of the product. |
language
OPTIONAL
|
string Language of the product (e.g., "en" or "ar"). By default, it is set to 'ar' |
currency
OPTIONAL
|
stringThe currency code (e.g., "QAR"). |
status
OPTIONAL
|
stringSpecifies the product's status within the store. When
adding a product to the store, you can customize how this product is
displayed in the store to enhance marketing efforts. There are various
available statuses:
(e.g., "Normal", "Sold", "New", "Discount", "Featured", "Private").
|
link
OPTIONAL
|
string A link related to the product. |
category
OPTIONAL
|
string Categories associated with the product. You can add multiple categories as a comma-separated string in the following format: "category1, category2, ... etc." |
setting
OPTIONAL
|
object Product settings containing: |
setting.is_public
OPTIONAL
|
booleanIndicates whether the product should be displayed in the store or not. By default, it is set to 'false,' and when you choose to display the product, its status will be set to "Normal" unless you change this status. |
setting.is_limited
OPTIONAL
|
booleanIndicates whether the product has a limited quantity. By default, it is set to 'false,' and it will be automatically changed to 'false' when you assign a value to 'max_count. |
setting.is_casheir
OPTIONAL
|
booleanIndicates whether the product will be displayed in the cashier interface or not. By default, it is set to 'false' |
setting.max_count
OPTIONAL
|
decimalIndicates the maximum available quantity of the product in the store. By default, null means unlimited quantity |
setting.max_order
OPTIONAL
|
integerindicates the maximum quantity that a customer can purchase of this product. By default, null means unlimited quantity |
Response
HTTP Status Code: 200 OK - The product's information has been successfully updated.
Response Schema: application/json | |
---|---|
status
|
string the status of response. SUCCESS |
product
|
object:
|
Otherwise if there was a problem with your request, you'll receive an error response such as 400 status code, and the response will include an error object describing why this request failed.
Response Schema: application/json | |
---|---|
status
|
string The status of response. |
error
|
object:
|
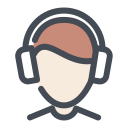
🛠️ Technical Support
- if you need any help you can check our help center, or contact support team