#Get Products API.
The following endpoint enables merchants to access information about their products, facilitating management and retrieval of product-related data. It supports filtering and pagination options, making it a valuable tool for managing product information efficiently.
https://api.fatora.io/v1/products
curl --location --request GET 'https://api.fatora.io/v1/products?page=1&page_size=20&status=online&SKU=KE-123&category=cat&is_chasier=true&is_public=ture&from=01-01-2024=&to=31-12-2024' \
--header "Authorization Bearer: eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1bmlxdWVfbmFtZSI6IjcyOTEyLTpyYW1pcmVzc2xhbkBnbWFpbC5jb20iLCJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9oYXNoIjoiOTExM2Y2OWEtMTYzMi00Nzg2LWE4NTctYmEzNWQ5YmEwMDc0IiwibmJmIjoxNzE5OTI0NDkzLCJleHAiOjE3NTEwMjg0OTMsImlhdCI6MTcxOTkyNDQ5M30.-rP1DT8LKk5jvBT_PpvaqFuUf_H6FhFiqAq9FM-34cw" \
--header 'Content-Type: application/json' \
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://api.fatora.io/v1/products?page=1&page_size=20&status=online&SKU=KE-123&category=cat&is_chasier=true&is_public=ture&from=01-01-2024=&to=31-12-2024',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_HTTPHEADER => array(
'Authorization: Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1bmlxdWVfbmFtZSI6IjcyOTEyLTpyYW1pcmVzc2xhbkBnbWFpbC5jb20iLCJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9oYXNoIjoiOTExM2Y2OWEtMTYzMi00Nzg2LWE4NTctYmEzNWQ5YmEwMDc0IiwibmJmIjoxNzE5OTI0NDkzLCJleHAiOjE3NTEwMjg0OTMsImlhdCI6MTcxOTkyNDQ5M30.-rP1DT8LKk5jvBT_PpvaqFuUf_H6FhFiqAq9FM-34cw',
'Content-Type: application/json'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
// Define the API endpoint URL.
string apiUrl = "https://api.fatora.io/v1/products?page=1&page_size=20&status=online&SKU=KE-123&category=cat&is_chasier=true&is_public=ture&from=01-01-2024=&to=31-12-2024";
// Create a WebRequest.
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(apiUrl);
// Set the request method to GET.
request.Method = "GET";
request.AddHeader("Authorization", "Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1bmlxdWVfbmFtZSI6IjcyOTEyLTpyYW1pcmVzc2xhbkBnbWFpbC5jb20iLCJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9oYXNoIjoiOTExM2Y2OWEtMTYzMi00Nzg2LWE4NTctYmEzNWQ5YmEwMDc0IiwibmJmIjoxNzE5OTI0NDkzLCJleHAiOjE3NTEwMjg0OTMsImlhdCI6MTcxOTkyNDQ5M30.-rP1DT8LKk5jvBT_PpvaqFuUf_H6FhFiqAq9FM-34cw");
// Set the request content type.
request.ContentType = "application/json";
try
{
// Get the response.
using (HttpWebResponse response = (HttpWebResponse)request.GetResponse())
{
if (response.StatusCode == HttpStatusCode.OK)
{
// Read the response data.
using (var responseStream = response.GetResponseStream())
using (var reader = new StreamReader(responseStream))
{
string responseContent = reader.ReadToEnd();
Console.WriteLine("API Response: " + responseContent);
}
}
else
{
Console.WriteLine("API Request failed with status code: " + response.StatusCode);
}
}
}
catch (WebException ex)
{
Console.WriteLine("API Request failed with an exception: " + ex.Message);
}
{
"status": "SUCCESS",
"products": [{
"id": 123,
"name": "Sample Item1",
"price": 19.99,
"sku": "SKU123",
"image": "https://example.com/sample-image.jpg",
"description": "This is a sample item description.",
"creation_date": "2023-09-30T12:00:00",
"currency": "QAR",
"category": ["Category1", "Category2"],
"qr_code": "https://api.qrserver.com/v1/create-qr-code/?data=sampleQRcode&size=50x50",
"link": "https://example.com/sample-link",
"status": "Active",
"language": "en",
"setting": {
"is_public": true,
"is_limited": false,
"is_casheir": true,
"max_count": 100.5,
"max_order": 10
}
},
{
"id": 4334,
"name": "Sample Item2",
"price": 19.99,
"sku": "SKU4334",
"image": "https://example.com/sample-image.jpg",
"description": "This is a sample item description.",
"creation_date": "2023-09-30T12:00:00",
"currency": "QAR",
"category": ["Category1", "Category2"],
"qr_code": "https://api.qrserver.com/v1/create-qr-code/?data=sampleQRcode&size=50x50",
"link": "https://example.com/sample-link",
"status": "Active",
"language": "en",
"setting": {
"is_public": true,
"is_limited": false,
"is_casheir": true,
"max_count": 100.5,
"max_order": 10
}
},
{
"id": 4354,
"name": "Sample Item3",
"price": 19.99,
"sku": "SKU4354",
"image": "https://example.com/sample-image.jpg",
"description": "This is a sample item description.",
"creation_date": "2023-09-30T12:00:00",
"currency": "QAR",
"category": ["Category1", "Category2"],
"qr_code": "https://api.qrserver.com/v1/create-qr-code/?data=sampleQRcode&size=50x50",
"link": "https://example.com/sample-link",
"status": "Active",
"language": "en",
"setting": {
"is_public": true,
"is_limited": false,
"is_casheir": true,
"max_count": 100.5,
"max_order": 10
}
}
]
,
"total": 203,
"page_size": 20,
"page": 1
}
Try it now: Get Products API
Header Parameters
Header | Value |
---|---|
Authorization Bearer: bearer_token
REQUIRED
|
obtain the bearer token from the Authorize endpoin. Read more information about Authentication. |
Content-Type
REQUIRED
|
application/json |
Query Parameters
Parameter | Description |
---|---|
q
OPTIONAL
|
string The quantity you want to use for filtering (if needed). |
status
OPTIONAL
|
string Status of products: "online," "offline," or "cashier" (if needed). |
page
OPTIONAL
|
int The page number for pagination. |
page_size
OPTIONAL
|
int The page size for pagination. |
from
OPTIONAL
|
DateTime The start date for filtering products created on or after this date. |
to
OPTIONAL
|
DateTime The end date for filtering products created on or before this date. |
Id
OPTIONAL
|
int Unique identifier for filtering products by their ID. |
sku
OPTIONAL
|
string Stock Keeping Unit (SKU) for filtering products. |
is_cashier
OPTIONAL
|
bool Boolean value for filtering products that are cashiers. |
is_public
OPTIONAL
|
bool Boolean value for filtering products that are public. |
category
OPTIONAL
|
string string value for filtering products based on their category. |
min
OPTIONAL
|
int Minimum value for filtering products. |
max
OPTIONAL
|
int Maximum value for filtering products. |
Response
HTTP Status Code: 200 OK - The products information has been successfully retrieved.
Response Schema: application/json | |
---|---|
status
|
string the status of response. SUCCESS |
products
|
array of product objects:
|
page
|
int the current page number. 1 |
page_size
|
int the number of items per page. 20 |
total
|
int the total number of products. 2 |
Otherwise if there was a problem with your request, you'll receive an error response such as 400 status code, and the response will include an error object describing why this request failed.
Response Schema: application/json | |
---|---|
status
|
string The status of response. |
error
|
object:
|
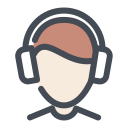
🛠️ Technical Support
- if you need any help you can check our help center, or contact support team