#Get Clients API.
The following endpoint enables merchants to access information about their clients, facilitating management and retrieval of client-related data. It supports filtering and pagination options, making it a valuable tool for managing client information efficiently.
https://api.fatora.io/v1/clients
curl --location --request GET 'https://api.fatora.io/v1/clients/?keyword=YourKeyword&email=ClientEmail&page=1&page_size=20' \
--header 'api_key: E4B73FEE-F492-4607-A38D-852B0EBC91C9' \
--header 'Content-Type: application/json' \
$curl = curl_init(); curl_setopt_array($curl, array(
CURLOPT_URL => 'https://api.fatora.io/v1/clients?keyword=YourKeyword&email=ClientEmail&page=1&page_size=20',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_HTTPHEADER => array(
'api_key: E4B73FEE-F492-4607-A38D-852B0EBC91C9',
'Content-Type: application/json'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
// Define the API endpoint URL.
string apiUrl = "https://api.fatora.io/v1/clients";
// Define the API key.
string apiKey = "E4B73FEE-F492-4607-A38D-852B0EBC91C9";
// Create a WebRequest.
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(apiUrl);
// Set the request method to GET.
request.Method = "GET";
// Set the API key header.
request.Headers.Add("api_key", apiKey);
// Set the request content type.
request.ContentType = "application/json";
try
{
// Get the response.
using (HttpWebResponse response = (HttpWebResponse)request.GetResponse())
{
if (response.StatusCode == HttpStatusCode.OK)
{
// Read the response data.
using (var responseStream = response.GetResponseStream())
using (var reader = new StreamReader(responseStream))
{
string responseContent = reader.ReadToEnd();
Console.WriteLine("API Response: " + responseContent);
}
}
else
{
Console.WriteLine("API Request failed with status code: " + response.StatusCode);
}
}
}
catch (WebException ex)
{
Console.WriteLine("API Request failed with an exception: " + ex.Message);
}
{
"status": "SUCCESS",
"clients": [
{
"id": 123,
"name": "Client Name 1",
"address": "Client Address 1",
"phone": "Client Phone 1",
"email": "[email protected]",
"notes":"Additional notes"
},
{
"id": 124,
"name": "Client Name 2",
"address": "Client Address 2",
"phone": "Client Phone 2",
"email": "[email protected]",
"notes":null
}
],
"total": 47,
"page_size": 20,
"page": 1
}
Try it now: Get Client API
Header Parameters
Header | Value |
---|---|
api_key
REQUIRED
|
Use the valid API key of your Fatora account. Read more information about Authentication. |
Content-Type
REQUIRED
|
application/json |
Query Parameters
Parameter | Description |
---|---|
keyword
OPTIONAL
|
string The keyword you want to use for filtering (if needed). |
email
OPTIONAL
|
string The email you want to filter (if needed). |
page
OPTIONAL
|
int The page number. |
page_size
OPTIONAL
|
int The page size. |
Response
HTTP Status Code: 200 OK - The clients information has been successfully retrieved.
Response Schema: application/json | |
---|---|
status
|
string the status of response. SUCCESS |
clients
|
array of objects:
|
page
|
int the current page number. 1 |
page_size
|
int the number of items per page. 20 |
total
|
int the total number of clients. 2 |
Otherwise if there was a problem with your request, you'll receive an error response such as 400 status code, and the response will include an error object describing why this request failed.
Response Schema: application/json | |
---|---|
status
|
string The status of response. |
error
|
object:
|
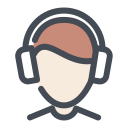
🛠️ Technical Support
- if you need any help you can check our help center, or contact support team