#Get Invoice API.
The following endpoint allows merchant to get invoice from their Fatora Platform account.
https://api.fatora.io/v1/invoices/{invoice_id}
curl --location --request GET 'https://api.fatora.io/v1/invoices/{invoice_id}' \
--header "Authorization: Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1bmlxdWVfbmFtZSI6IjcyOTEyLTpyYW1pcmVzc2xhbkBnbWFpbC5jb20iLCJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9oYXNoIjoiOTExM2Y2OWEtMTYzMi00Nzg2LWE4NTctYmEzNWQ5YmEwMDc0IiwibmJmIjoxNzE5OTI0NDkzLCJleHAiOjE3NTEwMjg0OTMsImlhdCI6MTcxOTkyNDQ5M30.-rP1DT8LKk5jvBT_PpvaqFuUf_H6FhFiqAq9FM-34cw" \
--header 'Content-Type: application/json' \
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://api.fatora.io/v1/invoices/{invoice_id}',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_HTTPHEADER => array(
'Authorization: Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1bmlxdWVfbmFtZSI6IjcyOTEyLTpyYW1pcmVzc2xhbkBnbWFpbC5jb20iLCJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9oYXNoIjoiOTExM2Y2OWEtMTYzMi00Nzg2LWE4NTctYmEzNWQ5YmEwMDc0IiwibmJmIjoxNzE5OTI0NDkzLCJleHAiOjE3NTEwMjg0OTMsImlhdCI6MTcxOTkyNDQ5M30.-rP1DT8LKk5jvBT_PpvaqFuUf_H6FhFiqAq9FM-34cw',
'Content-Type: application/json'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
// Define the API endpoint URL.
string apiUrl = "https://api.fatora.io/v1/invoices/{invoice_id}";
// Create a WebRequest.
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(apiUrl);
// Set the request method to GET.
request.Method = "GET";
request.AddHeader("Authorization", "Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1bmlxdWVfbmFtZSI6IjcyOTEyLTpyYW1pcmVzc2xhbkBnbWFpbC5jb20iLCJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9oYXNoIjoiOTExM2Y2OWEtMTYzMi00Nzg2LWE4NTctYmEzNWQ5YmEwMDc0IiwibmJmIjoxNzE5OTI0NDkzLCJleHAiOjE3NTEwMjg0OTMsImlhdCI6MTcxOTkyNDQ5M30.-rP1DT8LKk5jvBT_PpvaqFuUf_H6FhFiqAq9FM-34cw");
// Set the request content type.
request.ContentType = "application/json";
try
{
// Get the response.
using (HttpWebResponse response = (HttpWebResponse)request.GetResponse())
{
if (response.StatusCode == HttpStatusCode.OK)
{
// Read the response data.
using (var responseStream = response.GetResponseStream())
using (var reader = new StreamReader(responseStream))
{
string responseContent = reader.ReadToEnd();
Console.WriteLine("API Response: " + responseContent);
}
}
else
{
Console.WriteLine("API Request failed with status code: " + response.StatusCode);
}
}
}
catch (WebException ex)
{
Console.WriteLine("API Request failed with an exception: " + ex.Message);
}
{
"status": "SUCCESS",
"invoice":
{
"id": 2075,
"number": "INV567",
"currency": "QAR",
"terms_conditions": "Sample terms and conditions",
"note": null,
"date": "2023-10-24T10:21:00.9047758+02:00",
"status": "paid",
"type": "Detailed invoice",
"client": {
"id": 1307,
"name": "Client name",
"address": "Client address",
"phone": "Phone number",
"email": "[email protected]",
"notes": null
},
"title": "Sample Invoice",
"link": "https://example.com/sample-link",
"items": [
{
"id": 2376,
"description": "Sample invoice description",
"type": "Service Item",
"qty": 1.0,
"amount": 100.0
}
],
"payments": [
{
"id": 942,
"payment_date": "2023-10-10T00:00:00",
"transaction_id": "TXN123",
"amount": 100.0,
"note": "external",
"payment_type": "Credit",
"status": "Paid"
},
{
"id": 943,
"payment_date": "2023-10-10T00:00:00",
"transaction_id": "TXN456",
"amount": 50.0,
"note": "external",
"payment_type": "PayPal",
"status": "Paid"
}
],
"setting": {
"tax": false,
"discount": 0.0,
"discount_type": "",
"language": "ar",
"accepted_payment_methods": {
"card": true,
"bank_transfer": true,
"cash": true,
"paypal": true,
"e_signature": true
}
}
}
}
Try it now: Get Invoice API
Header Parameters
Header | Value |
---|---|
Authorization: Bearer bearer_token
REQUIRED
|
obtain the bearer token from the Authorize endpoin. Read more information about Authentication. |
Content-Type
REQUIRED
|
application/json |
Query Parameters
Parameter | Description |
---|---|
invoice_id
REQUIRED
|
intUnique identifier of the invoice to retrieved. |
Response
HTTP Status Code: 200 OK - The invoice information has been successfully retrieved.
Response Schema: application/json | |
---|---|
status
|
string the status of response. SUCCESS |
invoice
|
object item:
|
Otherwise if there was a problem with your request, you'll receive an error response such as 400 status code, and the response will include an error object describing why this request failed.
Response Schema: application/json | |
---|---|
status
|
string The status of response. |
error
|
object:
|
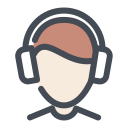
🛠️ Technical Support
- if you need any help you can check our help center, or contact support team