Standard Checkout API
Integrate Fatora Standard Checkout with your website to start accepting online payments from your clients.
Integration Steps:
Request a checkout URL from Fatora payment gateway.
The following endpoint is used for request a checkout URL in your (backend) server.
curl -X POST 'https://api.fatora.io/v1/payments/checkout' \
--header 'Content-Type: application/json' \
--header 'api_key: E4B73FEE-F492-4607-A38D-852B0EBC91C9' \
--data-raw '{
"amount":123.100,
"currency":"QAR",
"order_id":"123456",
"client" : {
"name" : "client",
"phone" : "+974XXXXXXXX",
"email" : "[email protected]"
},
"language":"en",
"success_url" : "http://domain.com/payments/success",
"failure_url" : "http://domain.com/payments/failure",
"fcm_token" : "XXXXXXXXX",
"save_token" : true,
"note": "some additional info"
}'
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://api.fatora.io/v1/payments/checkout',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_HTTPHEADER => array(
'Content-Type: application/json',
'api_key: E4B73FEE-F492-4607-A38D-852B0EBC91C9'
),
CURLOPT_POSTFIELDS =>'{
"amount":123.100,
"currency":"QAR",
"order_id":"123456",
"client" : {
"name" : "client",
"phone" : "+974XXXXXXXX",
"email" : "[email protected]"
},
"language":"en",
"success_url" : "http://domain.com/payments/success",
"failure_url" : "http://domain.com/payments/failure",
"fcm_token" : "XXXXXXXXX",
"save_token" : true,
"note": "some additional info"
}'
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
using (var httpClient = new HttpClient())
{
using (var request = new HttpRequestMessage(new HttpMethod("POST"), "https://api.fatora.io/v1/payments/checkout"))
{
request.Headers.Add("api_key", "E4B73FEE-F492-4607-A38D-852B0EBC91C9");
request.Content.Headers.ContentType = MediaTypeHeaderValue.Parse("application/json");
Payment payment = new Payment()
{
amount = 123.100,
currency = "QAR",
order_id = "123456",
client = new Client() {
name = "client",
phone = "+974XXXXXXXX",
email = "[email protected]"
},
language = "ar",
success_url = "http://domain.com/payments/success",
failure_url = "http://domain.com/payments/failure",
"fcm_token" = "XXXXXXXXX",
save_token = true,
note = "some additional info"
};
string payload = JsonConvert.SerializeObject(payment);
request.Content = new StringContent(payload);
var response = httpClient.SendAsync(request);
}
}
{
status:"SUCCESS",
"result": {
"checkout_url": "https://maktapp.credit/payments/checkoutpage?payment_id=XXXXXXXXXX"
} }
Try it now: Standard Checkout API
Header Parameters
Header | Value |
---|---|
api_key
REQUIRED
|
Use the valid API key of your Fatora account. Read more information about Authentication. |
Content-Type
REQUIRED
|
application/json |
Request Body
Parameter | Description |
---|---|
amount
REQUIRED
|
decimalIndicates the amount of the payment request. The dot is used as a decimal separator. |
currency
OPTIONAL
|
stringCurrency used for the order. Use the three-character ISO Standard Currency Codes e.g. USD, default is QAR
|
order_id
OPTIONAL
|
stringA unique identifier for order in your application |
client.name
OPTIONAL
|
stringThe client's full name. |
client.phone
REQUIRED-IF
|
stringThe client's phone number including country code. e.g. +974XXXXXXXX. It is required only if client.email does not provided. |
client.email
REQUIRED-IF
|
stringThe valid client's email. e.g. [email protected]. it is required only if client.phone does not provided. |
language
OPTIONAL
|
stringValue representing the browser language of checkout payment page. enum values [en, ar], default is en. |
success_url
OPTIONAL
|
stringIt is a valid URL to redirect your client
when successfully payment completion. e.g. http://domain.com/payments/success. If the URL is not provided, the redirection will be stopped at Fatora`s default Fatora success page. |
failure_url
OPTIONAL
|
string
It is a valid URL to redirect your client
when failure payment completion. e.g. http://domain.com/payments/success. If the URL is not provided, the redirection will be stopped at Fatora`s default Fatora failure page. |
fcm_token
OPTIONAL
|
string It is the registration token in Firebase Cloud Messaging, to receive message after payment completion. Read more information about Mobile Integration. |
save_token
OPTIONAL
|
booleanTo accept and save the payment card using a card token,
For more information read about
Tokenization Integration.
Possible values:
|
note
OPTIONAL
|
stringAdditional information about order, IT will appear in invoice title after successful payment |
Response
The successful request returns the HTTP 200 OK status code and a JSON response body that shows the checkout url to which you should redirect your client to fill his card data.
Response Schema: application/json | |
---|---|
status
|
string the status of response. SUCCESS |
result
|
objectThe URL of checkout page:
|
Otherwise if there was a problem with your request, you'll receive an error response such as 400 status code, and the response will include an error object describing why this request failed.
Response Schema: application/json | |
---|---|
status
|
string The status of response. |
error
|
object:
|
Redirect to the checkout page
Redirect your client to the checkout page using the URL which you have received in the response above, using either a server-side or client-side call.
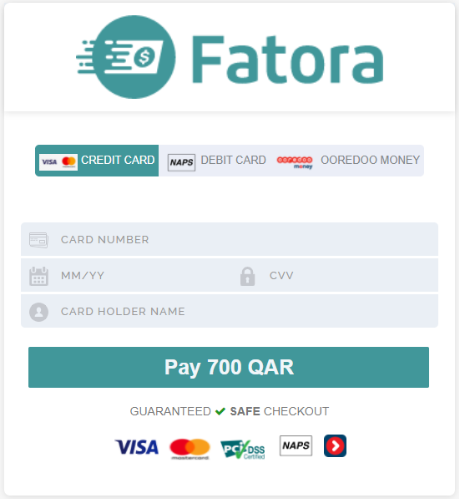
Payment completion
When your client completes the payment, the Fatora gateway will redirect to the default Fatora success | failure page, and if you pass success_url | failure_url at requesting Standard Checkout API, Fatora will redirect after 2 seconds to the (success | failure) page including order_id, transaction_id, and response_code in the query parameters.
Redirect to:
https://domain.com/payments/success?transaction_id=XXX&order_id=XXX&mode=XXX&
response_code=000&description=XXX
Redirect to:
https://domain.com/payments/failure?transaction_id=XXX&order_id=XXX&mode=XXX&
response_code=XXX&description=XXX
Parameters Description
Parameters | Description | |
---|---|---|
transaction_id
|
stringThe transaction id of payment issued from bank. You must keep this ID for future requests or to follow bank. | |
order_id
|
stringThe same unique identifier for order in your application which you sent on request checkout API. | |
card_token
|
string
It is an unique identifier will be used in collect new payment API. It
returns only when passed
save_token = true .
|
|
mode
|
stringThe value of your integration mode, enum values ["test", "live"]. | |
response_code
|
stringThe value of response code | |
description
|
stringAdditional information about transaction. |
Webhook
Webhook is used for asynchronous events after payment completion whether it has been done successfully or failure. Fatora gateway trigger your webhook, passing parameters to URL in this form:
https://domain.com/payments/webhook?
transaction_id=XXXXXX&order_id=XXXXXX&mode=XXX&
response_code=XXX&description=XXXXXXXXXX
Webhook Configuration:
In order to configure the webhook, provide your webhook URL. Please follow the below steps:
-
Log in to your Fatora account.
-
Choose the Integration from the sidebar navigation, then click on Settings.
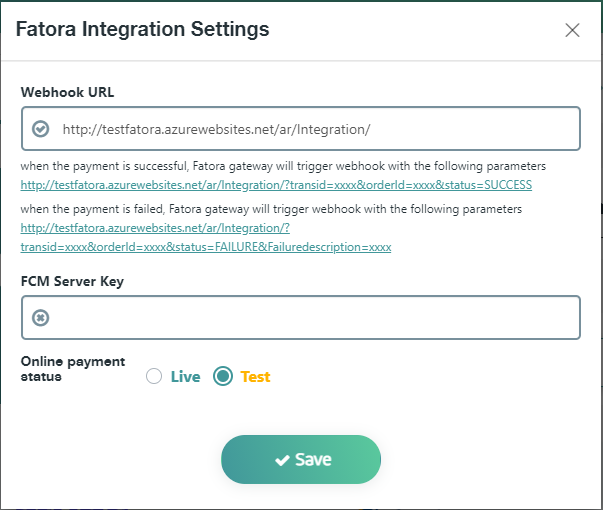