#Update Client API.
The following endpoint allows merchant to update client in their Fatora Platform account.
https://api.fatora.io/v1/clients/{client_id}
curl --location --request PUT 'https://api.fatora.io/v1/clients/1024' \
--header "Authorization: Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1bmlxdWVfbmFtZSI6IjcyOTEyLTpyYW1pcmVzc2xhbkBnbWFpbC5jb20iLCJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9oYXNoIjoiOTExM2Y2OWEtMTYzMi00Nzg2LWE4NTctYmEzNWQ5YmEwMDc0IiwibmJmIjoxNzE5OTI0NDkzLCJleHAiOjE3NTEwMjg0OTMsImlhdCI6MTcxOTkyNDQ5M30.-rP1DT8LKk5jvBT_PpvaqFuUf_H6FhFiqAq9FM-34cw" \
--header 'Content-Type: application/json' \
--data-raw '{
"name": "Client Name",
"address": "Client Address",
"phone": "Client Phone",
"email": "[email protected]",
"notes": "Additional notes"
}'
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://api.fatora.io/v1/clients/1024',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'PUT',
CURLOPT_POSTFIELDS =>'{
"name": "Client Name",
"address": "Client Address",
"phone": "Client Phone",
"email": "[email protected]",
"notes": "Additional notes"
}',
CURLOPT_HTTPHEADER => array(
'Authorization: Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1bmlxdWVfbmFtZSI6IjcyOTEyLTpyYW1pcmVzc2xhbkBnbWFpbC5jb20iLCJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9oYXNoIjoiOTExM2Y2OWEtMTYzMi00Nzg2LWE4NTctYmEzNWQ5YmEwMDc0IiwibmJmIjoxNzE5OTI0NDkzLCJleHAiOjE3NTEwMjg0OTMsImlhdCI6MTcxOTkyNDQ5M30.-rP1DT8LKk5jvBT_PpvaqFuUf_H6FhFiqAq9FM-34cw',
'Content-Type: application/json'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
// Define the API endpoint URL.
string apiUrl = "https://api.fatora.io/v1/clients/1024";
var client = new Client
{
name = "Client Name",
phone = "Client Phone",
email = "[email protected]",
address = "Client Address",
Note = "Additional notes"
};
// Serialize the Client object to JSON.
string jsonPayload = JsonConvert.SerializeObject(client);
// Create a WebRequest.
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(apiUrl);
// Set the request method to PUT.
request.Method = "PUT";
request.AddHeader("Authorization", "Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1bmlxdWVfbmFtZSI6IjcyOTEyLTpyYW1pcmVzc2xhbkBnbWFpbC5jb20iLCJodHRwOi8vc2NoZW1hcy54bWxzb2FwLm9yZy93cy8yMDA1LzA1L2lkZW50aXR5L2NsYWltcy9oYXNoIjoiOTExM2Y2OWEtMTYzMi00Nzg2LWE4NTctYmEzNWQ5YmEwMDc0IiwibmJmIjoxNzE5OTI0NDkzLCJleHAiOjE3NTEwMjg0OTMsImlhdCI6MTcxOTkyNDQ5M30.-rP1DT8LKk5jvBT_PpvaqFuUf_H6FhFiqAq9FM-34cw");
// Set the request content type.
request.ContentType = "application/json";
// Convert the JSON payload to bytes.
byte[] data = Encoding.UTF8.GetBytes(jsonPayload);
// Set the content length.
request.ContentLength = data.Length;
// Get the request stream and write the JSON data to it.
using (var stream = request.GetRequestStream())
{
stream.Write(data, 0, data.Length);
}
try
{
// Get the response.
using (HttpWebResponse response = (HttpWebResponse)request.GetResponse())
{
if (response.StatusCode == HttpStatusCode.OK)
{
// Read the response data.
using (var responseStream = response.GetResponseStream())
using (var reader = new StreamReader(responseStream))
{
string responseContent = reader.ReadToEnd();
Console.WriteLine("API Response: " + responseContent);
}
}
else
{
Console.WriteLine("API Request failed with status code: " + response.StatusCode);
}
}
}
catch (WebException ex)
{
Console.WriteLine("API Request failed with an exception: " + ex.Message);
}
{
"status": "SUCCESS",
"client": {
"id": 1024,
"name":"Client Name",
"phone":"Client Phone",
"email":"[email protected]",
"address":"Client Address",
"note": "Additional notes"
}
}
Try it now: Update Client API
Header Parameters
Header | Value |
---|---|
Authorization: Bearer bearer_token
REQUIRED
|
obtain the bearer token from the Authorize endpoin. Read more information about Authentication. |
Content-Type
REQUIRED
|
application/json |
Query Parameters
Parameter | Description |
---|---|
client_id
REQUIRED
|
intUnique identifier of the client to update. |
Request Body
Parameter | Description |
---|---|
name
Optional
|
stringThe client`s full name |
phone
OPTIONAL
|
stringThe client's phone number. |
email
REQUIRED
|
stringThe client's email address. |
address
OPTIONAL
|
stringThe client's address. |
notes
OPTIONAL
|
stringAdditional information. |
Response
HTTP Status Code: 200 OK - The client's information has been successfully updated.
Response Schema: application/json | |
---|---|
status
|
string the status of response. SUCCESS |
client
|
object:
|
Otherwise if there was a problem with your request, you'll receive an error response such as 400 status code, and the response will include an error object describing why this request failed.
Response Schema: application/json | |
---|---|
status
|
string The status of response. |
error
|
object:
|
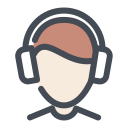
🛠️ Technical Support
- if you need any help you can check our help center, or contact support team